In today’s digital age, efficiently managing and annotating PDF documents is more critical than ever. Professionals and students alike need tools that allow for easy markup, collaboration, and feedback on PDF files. React, a popular JavaScript library, provides an excellent foundation for building robust PDF annotation tools that enhance productivity and streamline workflows.
PDFs are widely used for their portability and consistency across different devices and platforms. However, without proper annotation tools, interacting with these documents can be cumbersome. This is where PDF annotation tools come in, offering capabilities to highlight, comment, and mark up documents efficiently. Using React for building these tools brings an additional layer of efficiency and ease due to its robust framework and active community support.
Part 1. Understanding React PDF Annotation
What is React PDF Annotation?
React PDF annotation involves using React to create dynamic and interactive annotations on PDF documents. By leveraging React’s capabilities, developers can build sophisticated tools that allow users to highlight text, add comments, draw shapes, and more, all within their web applications.
React PDF annotation is the process of adding interactive features to PDF documents within a React-based web application. This includes functionalities like text highlighting, commenting, and drawing, which enhance the usability and interactivity of PDF files. React's component-based architecture allows for the creation of modular and maintainable code, making it easier to add and update features as needed.
The Benefits of Using React for PDF Annotation
Using React for PDF annotation offers several advantages:
- Modular Development: React’s component-based structure allows developers to build and maintain complex applications efficiently.
- High Performance: React’s virtual DOM ensures that updates and rendering are fast and efficient, providing a smooth user experience.
- Flexibility: React integrates well with other libraries, making it possible to customize and extend functionalities as needed.
By leveraging React for PDF annotation, developers can create applications that are not only powerful, but also easy to maintain and scale. The modular nature of React components allows for building features incrementally, ensuring that the application remains flexible and adaptable to new requirements.
Part 2. Why Choose React for PDF Annotation?
Leveraging React's Capabilities
React excels in managing complex states and user interactions, which are essential for an interactive PDF annotation tool. Its declarative nature simplifies the development process, allowing developers to focus on creating functional components without worrying about direct DOM manipulation.
React is particularly well-suited for handling dynamic user interfaces that require real-time updates, such as those needed for PDF annotation. Its ability to manage state efficiently ensures that user interactions are smooth and responsive, providing an optimal user experience.
Integration with Libraries and Tools
React’s ecosystem includes numerous libraries that can enhance PDF annotation capabilities. For example, react-pdf helps with rendering PDFs, while pdf-lib provides tools for creating and modifying PDFs. These integrations enable developers to build powerful and feature-rich annotation tools.
The rich ecosystem of React makes it possible to integrate various third-party libraries that add specialized functionalities to your PDF annotation tool. This flexibility allows developers to create comprehensive solutions tailored to specific needs without having to reinvent the wheel.
Performance Benefits
React’s efficient rendering through the virtual DOM reduces the load on the browser, ensuring smooth performance even with extensive annotations and interactions. This performance boost is crucial for maintaining a responsive and user-friendly application.
The virtual DOM is a key feature of React that enhances performance by minimizing direct manipulations of the actual DOM. This leads to faster updates and rendering, which is particularly beneficial for applications that involve frequent and complex interactions, such as PDF annotation tools.
Part 3. Getting Started with React PDF Annotation
Prerequisites
Before starting, ensure you have:
- js and npm installed
- Basic knowledge of React and JavaScript (ES6+)
Having these prerequisites in place will ensure a smoother development process. A solid understanding of React and JavaScript is essential for building a functional and efficient PDF annotation tool.
Installation Guide
- Create a New React Project: Open your terminal and run:

- Install Essential Libraries:

By following these steps, you'll set up a basic React project with the necessary libraries for PDF annotation. This setup provides a strong foundation for building your tool.
Essential Libraries
- @react-pdf-viewer/core: For rendering PDFs in React.
- @react-pdf-viewer/annotation: For adding annotation features.
- pdf-lib: For creating and modifying PDF documents.
These libraries offer the core functionality needed to build a comprehensive PDF annotation tool. They provide robust features for rendering and interacting with PDF documents within a React application.
Part 4. Building Your First React PDF Annotation Tool
Project Setup and Configuration
Begin by structuring your React application. Create components dedicated to rendering PDFs and handling annotations.
Creating Components
1. PDF Viewer Component:
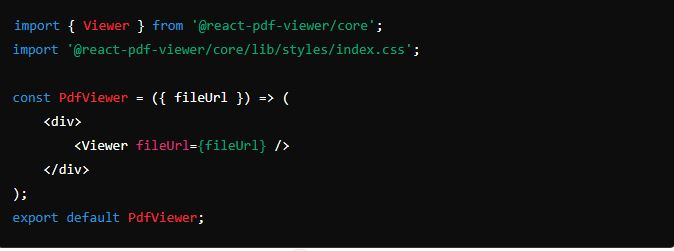
2. Annotation Component:
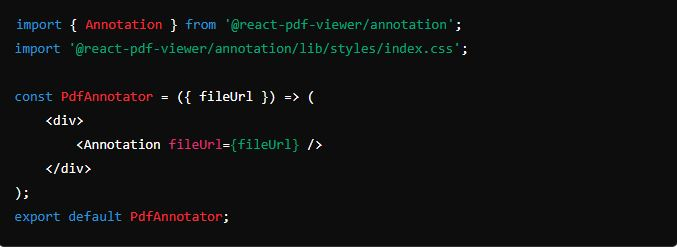
Core Annotation Features
Implement basic features such as text highlighting and commenting. Ensure these features are user-friendly and intuitive.
Building a functional PDF annotation tool involves setting up components for rendering the PDF and adding annotations. The example components above provide a starting point for integrating PDF viewing and annotation capabilities into your React application.
Part 5. Expanding Functionality with Advanced Features
Text Highlighting
Use pdf-lib to enable users to highlight important text within the PDF. This is crucial for reviewing and studying documents.
Text highlighting allows users to emphasize important sections of the document. This feature is essential for reviewing and studying documents, making it a key component of any annotation tool.
Commenting and Note-Taking
Allow users to add comments and notes to specific sections of the PDF. This enhances collaboration and feedback mechanisms.
Commenting and note-taking features enable users to provide detailed feedback and annotations. This functionality is crucial for collaborative environments where multiple users need to review and discuss documents.
Drawing Tools
Provide tools for drawing shapes like rectangles, circles, and free-form lines. This adds another layer of functionality for users needing detailed annotations.
Drawing tools allow for more expressive and detailed annotations. Users can highlight sections, draw attention to specific areas, and provide visual feedback using shapes and free-form lines.
Part 6. Customization: Tailoring Annotations to User Preferences
Styles and Colors
Offer options to customize annotation styles and colors. Personalization enhances the user experience by allowing individuals to use the tool in a way that suits their needs.
Customization enhances the user experience by allowing users to tailor the tool to their preferences. Offering options for different styles and colors makes the annotation process more enjoyable and efficient.
Toolbar Layout
Allow users to personalize the toolbar layout, ensuring they can access frequently used tools quickly. This customization can significantly boost productivity.
A customizable toolbar ensures that users can access their most-used tools with ease. This personalization can significantly enhance productivity and user satisfaction.
Saving Preferences
Implement features to save user preferences, ensuring a consistent experience across sessions. This makes the tool more user-friendly and tailored to individual needs.
Saving user preferences provides a consistent experience across sessions. This feature ensures that users can pick up where they left off without having to reset their preferences each time.
Part 7. Efficient Storage and Retrieval of Annotations
Local Storage
Store annotations locally in the browser using local storage. This provides quick access and retrieval of annotations without the need for a server.
Local storage is a convenient solution for saving annotations for individual users. It allows for quick access and is easy to implement, making it ideal for single-user applications.
Server-Side Storage
For more reliable and scalable storage, implement server-side solutions using APIs to save and retrieve annotations.
Server-side storage provides a more robust solution for applications with multiple users or large datasets. It ensures that annotations are reliably stored and can be accessed from any device.
Database Integration
Use databases for scalable and long-term storage of annotations. This is especially beneficial for applications with numerous users.
Database integration offers scalable and long-term storage solutions. This approach is ideal for enterprise applications where data integrity and reliability are paramount.
Part 8. Handling Various PDF Formats and Versions
Compatibility
Ensure your tool supports various PDF standards and versions to accommodate a wide range of documents. Compatibility is key for a versatile annotation tool.
Compatibility with different PDF standards is crucial for a versatile annotation tool. This ensures that users can work with any PDF document, regardless of its format or version.
Common Issues
Address common format-related issues to ensure smooth operation. Provide solutions and workarounds for users encountering these issues.
Handling format-related issues is essential for a seamless user experience. By addressing common problems, you can ensure that your tool works smoothly with all types of PDF documents.
PDF.js for Rendering
Use PDF.js for robust PDF rendering. It supports a wide range of PDF features, making it an excellent choice for building comprehensive annotation tools.
PDF.js is a powerful library for rendering PDFs in web applications. Its versatility and robust feature set make it an excellent choice for building a comprehensive PDF annotation tool.
Part 9. Optimizing Performance for Smooth User Experience
Load Time Reduction
Implement techniques such as lazy loading to reduce load times. This ensures a smooth and responsive user experience, particularly for large PDFs.
Reducing load times is critical for a smooth user experience. Techniques like lazy loading can help minimize initial load times, making the tool more responsive.
Efficient Rendering
Optimize the rendering process for large PDFs by implementing strategies such as page-by-page rendering. This approach improves performance and ensures smoother navigation.
Efficient rendering strategies are essential for handling large PDF documents. By rendering pages on demand, you can improve performance and ensure a smoother experience.
Performance Enhancements
Use performance enhancement techniques like lazy loading to improve overall efficiency. Loading resources as needed can significantly boost the application's responsiveness.
Lazy loading is an effective technique for enhancing performance. By loading resources only when needed, you can reduce the initial load time and improve the overall user experience.
Part 10. Enhancing Functionality with Third-Party Libraries
Popular Libraries
Explore libraries like annotator.js and pdf-lib to enhance your tool’s capabilities. These libraries provide additional functionality and features that can be seamlessly integrated into your React application.
Third-party libraries can add valuable functionality to your PDF annotation tool. By leveraging these libraries, you can enhance your tool’s capabilities without starting from scratch.
Library Integration
Provide detailed instructions for integrating these libraries. Clear documentation ensures that the integration process is smooth and straightforward.
Integrating third-party libraries can significantly enhance your tool’s functionality. A clear and detailed guide will help ensure a smooth integration process.
Pros and Cons
Evaluate the advantages and disadvantages of each library to choose the best tools for your needs. Consider factors such as ease of integration, functionality, and community support.
Part 11. Designing for Exceptional User Experience
Intuitive Interface
Design an intuitive and user-friendly interface. Ensure that all features are easily accessible and straightforward to use.
An intuitive and user-friendly interface is important for a positive user experience. Design your tool with the user in mind, ensuring that all features are handy to use.
Accessibility Features
Incorporate accessibility features to make your tool usable by everyone, including those with disabilities. This comprises keyboard navigation and screen reader support.
User Feedback
Gather feedback from users to continuously improve your tool. Implement changes based on this feedback to enhance user satisfaction and usability.
User feedback is invaluable for improving your tool. Collect and incorporate feedback to ensure that your tool meets the needs of your users.
Part 12. Testing and Debugging Your Annotation Tool
Common Issues
Addressing common issues and providing solutions helps users troubleshoot problems. This ensures a streamlined and reliable user experience.
Debugging Techniques
Effective debugging techniques are essential for maintaining a reliable tool. Use tools like Chrome DevTools to identify and fix bugs efficiently.
Testing Tools
Thorough testing is crucial for ensuring the reliability of your tool. Use tools like Jest and Enzyme and follow best practices to ensure your tool is robust and bug-free.
Part 13. Preparing for Deployment
Production Preparation
Preparing for deployment involves following best practices to ensure a smooth transition to production. Optimize performance, ensure security, and conduct thorough testing to guarantee a successful deployment.
Deployment Strategies
Choosing the right deployment strategy is critical for the success of your application. Consider factors like scalability, reliability, and ease of maintenance when planning your deployment.
Post-Deployment Monitoring
Post-deployment monitoring is essential for maintaining a smooth operation. Quickly addressing any issues that arise ensures that your users have a positive experience.
Part 14. Ensuring Security in Your PDF Annotation Tool
Data Protection
Implement encryption to protect user data. Ensuring that sensitive information remains secure is crucial for any application.
Authentication and Authorization
Use robust authentication and authorization mechanisms to prevent unauthorized access. This adds an extra layer of security to your tool.
Regular Updates
Keep all dependencies updated to prevent security vulnerabilities. Regular updates ensure your application remains secure and up-to-date.
Part 15. Looking Ahead: The Future of React PDF Annotation
Emerging Trends
Staying informed about emerging trends ensures that your tool remains relevant and competitive. Keep an eye on new developments in PDF annotation technology.
Upcoming Technologies
Keep an eye on upcoming technologies that could enhance your PDF annotation tool. Staying ahead of the curve ensures your tool remains competitive.
Future Predictions
Understanding predictions for the future of document management helps you plan for long-term success. Keep yourself informed about trends and developments in the field.
Conclusion
React PDF annotation offers a powerful solution for managing and enhancing PDF documents. By leveraging React, you can create a seamless and intuitive experience for annotating PDFs. Start building your React PDF annotation tool today and transform the way you work with digital documents.
While building your own React-based PDF annotation tool can provide a tailored solution, using a specialized product like AfirstSoft PDF Annotator can significantly streamline the process. AfirstSoft PDF Annotator is designed to meet all your PDF annotation needs, offering a wide range of features that are easy to use and highly effective.
Start using AfirstSoft PDF Annotator today to transform the way you interact with digital documents. Enhance your productivity, improve collaboration, and streamline your workflows with this powerful tool. Embrace the future of document management with AfirstSoft PDF Annotator and experience the difference it can make in your daily tasks.
FAQs
1. How do I set up a React PDF annotation tool?
Start by initializing a React project and installing necessary libraries like @react-pdf-viewer/core and pdf-lib. Follow our step-by-step guide to set up and configure your tool.
2. What are the benefits of using React for PDF annotation?
React offers a modular architecture, high performance through the virtual DOM, and seamless integration with other libraries. This makes it an ideal choice for building robust PDF annotation tools.
3. Can I customize the annotations in my React PDF tool?
Yes, you can customize annotation styles and colors, personalize the toolbar layout, and save user preferences for a tailored experience.
4. How can I save and load annotations?
Annotations can be saved locally using browser storage or server-side for enhanced reliability. Integrating with databases allows for scalable storage solutions.
5. What libraries can enhance my React PDF annotation tool?
Libraries like react-pdf, pdf-lib, and annotator.js offer additional functionality and features that can enhance your PDF annotation tool.
6. What security measures should I take for my React PDF annotation tool?
Implement data encryption, robust authentication and authorization, and regularly update dependencies to ensure security and prevent vulnerabilities.
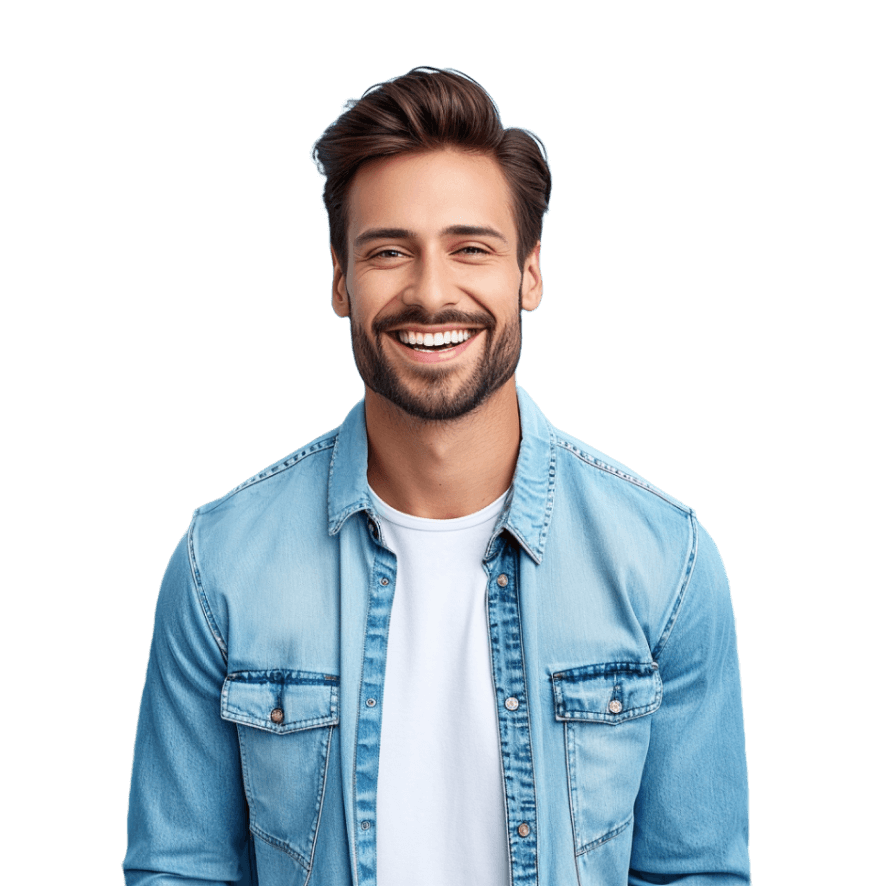
John Smith
Editor-in-Chief
With 10 years of experience in the office industry, John Smith is a tech enthusiast and seasoned copywriter. He likes sharing insightful product reviews, comparisons, and etc.
View all Articles >